In this simple project we will create a basic barometer using an MS5611 sensor
You can find out more about this sensor at http://www.te.com/usa-en/product-CAT-BLPS0036.html , the easiest way to use this sensor is using a module like the one below
MS5611 Features
- High resolution module, 10 cm
- Fast conversion down to 1 ms
- Low power, 1 µA (standby < 0.15 µA)
- QFN package 5.0 x 3.0 x 1.0 mm3
- Supply voltage 1.8 to 3.6 V
- Integrated digital pressure sensor (24 bit ΔΣ ADC)
- Operating range: 10 to 1200 mbar, -40 to +85 °C
- I2C and SPI interface up to 20 MHz
- No external components (Internal oscillator)
- Excellent long term stability
Layout
An easy device to connect to an Arduino
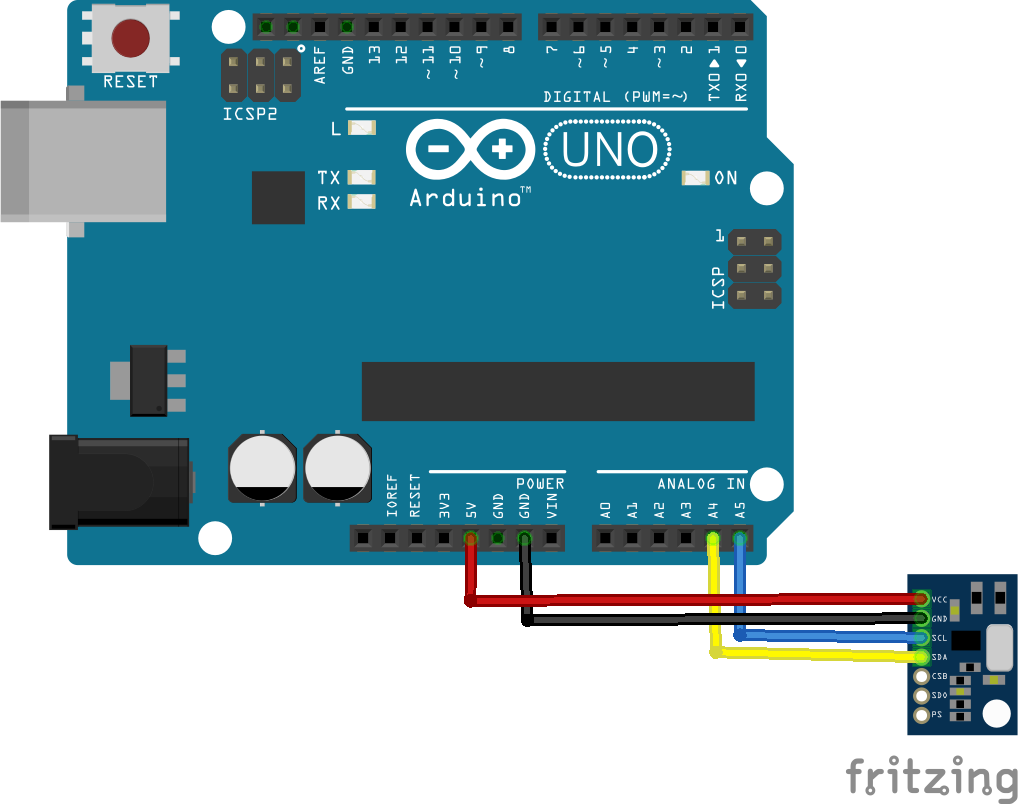
arduino and ms5611
Code
We use the https://github.com/jarzebski/Arduino-MS5611 library, the code below is slightly trimmed down
[codesyntax lang=”cpp”]
#include <Wire.h>
#include <MS5611.h>
MS5611 ms5611;
double referencePressure;
void setup()
{
Serial.begin(9600);
// Initialize MS5611 sensor
Serial.println(“Initialize MS5611 Sensor”);
while(!ms5611.begin())
{
Serial.println(“Could not find a valid MS5611 sensor, check wiring!”);
delay(500);
}
// Get reference pressure for relative altitude
referencePressure = ms5611.readPressure();
}
void loop()
{
// Read true temperature & Pressure
double realTemperature = ms5611.readTemperature();
long realPressure = ms5611.readPressure();
// Calculate altitude
float absoluteAltitude = ms5611.getAltitude(realPressure);
float relativeAltitude = ms5611.getAltitude(realPressure, referencePressure);
Serial.print(“Temp = “);
Serial.print(realTemperature);
Serial.println(” *C”);
Serial.print(“Pressure = “);
Serial.print(realPressure);
Serial.println(” Pa”);
Serial.print(“relativeAltitude = “);
Serial.print(relativeAltitude);
Serial.println(” m”);
delay(1000);
}
[/codesyntax]
Output
Open the serial monitor and you will see something like this
Temp = 22.59 *C
Pressure = 101749 Pa
relativeAltitude = 0.17 m
Temp = 22.59 *C
Pressure = 101751 Pa
relativeAltitude = 0.00 m
Temp = 22.59 *C
Pressure = 101755 Pa
relativeAltitude = -0.33 m
Temp = 22.60 *C
Pressure = 101757 Pa
relativeAltitude = -0.50 m
Ideally you could quite easily display these reading on an LCD display or even to a cloud server
Link
GY-63 MS5611-01BA03 Precision MS5611 Atmospheric Pressure Sensor Module Height Sensor Module