In this project we will create a basic gas sensor detector, we will use the CCS811 gas sensor and connect this to an LCD keypad shield.
Lets look at the gas sensor first.
The CCS811 is a low-power digital gas sensor solution, which integrates a gas sensor solution for detecting low levels of VOCs typically found indoors, with a microcontroller unit (MCU) and an Analog-to-Digital converter to monitor the local environment and provide an indication of the indoor air quality via an equivalent CO2 or TVOC output over a standard I2C digital interface.
Features
Integrated MCU
On-board processing
Standard digital interface
Optimised low power modes
IAQ threshold alarms
Programmable baseline
2.7mm x 4.0mm LGA package
Low component count
Proven technology platform
Specs
Interface | I²C |
---|---|
Supply Voltage [V] | 1.8 to 3.6 |
Power Consumption [mW] | 1.2 to 46 |
Dimension [mm] | 2.7 x 4.0 x 1.1 LGA |
Ambient Temperature Range [°C] | -40 to 85 |
Ambient Humidity Range [% r.h.] | 10 to 95 |
Parts List
I used the following parts for this project
Description | Link |
Arduino Uno | 1pcs UNO R3 CH340G+MEGA328P for Arduino UNO R3 (NO USB CABLE) |
LCD Keypad shield | 1PCS LCD Keypad Shield LCD 1602 Module Display For Arduino |
SHT31 sensor | CJMCU-811 CCS811 Air Quality Gas Sensor |
connecting wire | 40pcs Dupont jumper wire cable 30cm male to male,female to female,male to female Dupont jump wire line 2.54mm breadboard cable |
Schematics/Layout
Remember and connect the WAKE pin of the module to gnd
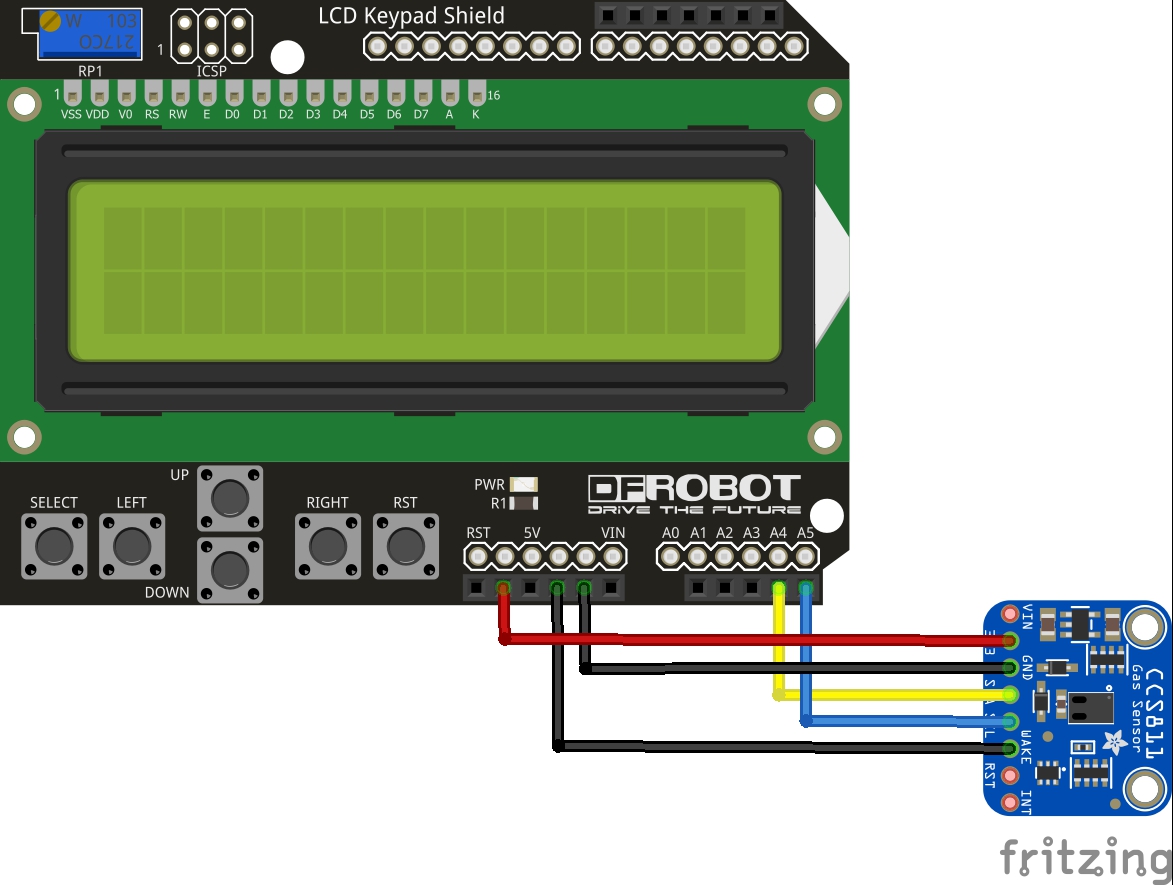
lcd shield and ccs811 gas sensor layout
Code
Again we use a library – its an adafruit one and you can add this using the Arduino library manager
[codesyntax lang=”cpp”]
#include <Wire.h> #include <LiquidCrystal.h> #include "Adafruit_CCS811.h" //setup for the LCD keypad shield LiquidCrystal lcd(8, 9, 4, 5, 6, 7); Adafruit_CCS811 ccs; void setup() { //init serial for some debug Serial.begin(9600); Serial.println("CCS811 test"); //init CCS811 sensor if(!ccs.begin()) { Serial.println("Failed to start sensor! Please check your wiring."); while(1); } //calibrate temperature sensor while(!ccs.available()); float temp = ccs.calculateTemperature(); ccs.setTempOffset(temp - 25.0); //init LCD lcd.begin(16,2); //line 1 - temperature lcd.setCursor(0,0); lcd.print("Temp = "); //line 2 - humidity lcd.setCursor(0,1); lcd.print("Hum = "); } void loop() { //display temperature on lcd if(ccs.available()) { float temp = ccs.calculateTemperature(); if(!ccs.readData()) { lcd.setCursor(0,0); lcd.print("CO2: "); lcd.print(ccs.geteCO2()); lcd.print(" ppm"); lcd.setCursor(0,1); lcd.print("TVOC: "); lcd.print(ccs.getTVOC()); } else { Serial.println("ERROR!"); while(1); } } delay(500); }
[/codesyntax]
Output
In this short video we will show what you can expect to see on the LCD.
Links